I’m pleased to announce the immediate availability of API Platform 2.4 beta! This new version is a huge one, that comes with a large set of new features.
For newcomers, API Platform is a full-stack framework to develop in a breath high quality API-driven projects. Among other (lower level) libraries, API Platform provides:
- a modular and fully-featured API library built on top of the Symfony components
- a beautiful admin interface dynamically generated from the API specification, built using React and React Admin
- a client generator to scaffold React/Redux, React Native and Vue.js apps from the API spec
API Platform supports modern REST formats (JSON-LD/Hydra, JSONAPI, OpenAPI, HAL…) as well as GraphQL.
To create your initial project, you just have to describe the public structures of the data to expose through the API. API Platform will take care of exposing the web API and bootstrapping the clients to consume it: get started with API Platform.
Version 2.4 introduces a lot of very interesting new features. Here is the curated list:
- Read and write support for MongoDB, the reference document database, including a lot of useful filters
- Read support for Elasticsearch, the open source search and analytics engine, including filters for advanced search
- Automatic “push” of updated resources from the server to the clients using the brand new Mercure protocol
- Integration with the Symfony Messenger component to easily implement the CQRS pattern and to handle messages asynchronously (using brokers such as RabbitMQ, Apache Kafka, Amazon SQS or Google PubSub)
- Ability to leverage the “Server Push” feature of HTTP/2 to preemptively send the relations of a requested resource to the client
- Automatic availability of list filters in the React-based admin when a corresponding one is available API-side
- Full compatibility with the version 3 of the OpenAPI specification format (formerly known as Swagger), and integration of the beautiful ReDoc documentation generator
- Improved DTOs support
- Per resource configuration of HTTP cache headers
- Ability to easily use the Sunset HTTP header to advertise the removal date of deprecated endpoints
The full changelog is available on GitHub.
Let’s dive into these new features!
MongoDB support
MongoDB is one of the most popular NoSQL database. Native support for MongoDB was the most requested, and the most awaited API Platform feature! Sam Van der Borght started the work in March 2016. In July 2017 the Pull Request has been ported to API Platform v2 by Pablo Godinez helped by Hamza Amrouche (API Platform Core Team), Alexandre Delplace and others. Finally, since August 2018 Alan Poulain (API Platform Core Team and author of the GraphQL subsystem) produced a huge effort to finish and polish the patch.
With 114 commits and 234 files changed over almost 3 years. This is one of the biggest contributions to the project.
The MongoDB integration relies on Doctrine MongoDB ODM 2.0 (currently in beta). To enable this feature, just install and configure DoctrineMongoDBBundle. API Platform will autodetect it. Then, create a class mapped with MongoDB, and mark it as an API resource:
namespace App\Document;
use ApiPlatform\Core\Annotation\ApiResource;
use Doctrine\ODM\MongoDB\Mapping\Annotations as ODM;
/**
* @ApiResource
* @ODM\Document
*/
class Book
{
/**
* @ODM\Id(strategy="INCREMENT", type="integer")
*/
public $id;
/**
* @ODM\Field(type="string")
*/
public $title;
}
The support for MongoDB leverages the flexibility of API Platform: it has been implemented as a data provider and a data persister. Relations, pagination as well as boolean, date, numeric, order, range and search filters are also supported!
A big thanks to all contributors of this amazing feature, and to Andreas Braun, the maintainer of Doctrine MongoDB ODM, for the in-depth reviews!
Elasticsearch support
Elasticsearch is another very popular open-source data store. It allows to perform full-text searches and advanced analyzes on very large datasets. Orange has sponsored the development of an Elasticsearch data provider for API Platform, as well as some interesting search filters. The implementation has been realized by Baptiste Meyer (API Platform Core Team). Thanks to Orange, this feature is now available for everybody in API Platform 2.4.
To enable and configure the Elasticsearch support, refer to the official documentation. Then, a simple resource class corresponding to an Elasticsearch index is enough to benefit from the full power of API Platform:
namespace App\Model;
use ApiPlatform\Core\Annotation\ApiProperty;
use ApiPlatform\Core\Annotation\ApiFilter;
use ApiPlatform\Core\Annotation\ApiResource;
use ApiPlatform\Core\Bridge\Elasticsearch\DataProvider\Filter\MatchFilter;
/**
* @ApiResource
*/
class Tweet
{
/**
* @ApiProperty(identifier=true)
*
* @var string
*/
public $id;
/**
* @var User
*/
public $author;
/**
* @var \DateTimeInterface
*/
public $date;
/**
* @ApiFilter(MatchFilter::class)
*
* @var string
*/
public $message;
}
Then, you can use an URL such as /tweets?message=foo
to search using Elasticsearch.
Keep in mind that it’s your responsibility to populate your Elastic index. To do so, you can use Logstash, a custom data persister or any other mechanism that fits for your project (such as an ETL).
Baptiste also took this opportunity to improve the code handling the pagination. It is now a generic class used by all native data providers (Doctrine ORM, MongoDB and Elasticsearch), that you can reuse in your own.
Read and improve the Elasticsearch related documentation.
Real time update of client with Mercure
Mercure is a brand new protocol built on top of HTTP/2 and Server-sent Events (SSE). It’s a modern and high-level alternative to WebSocket (WebSocket is not compatible with HTTP/2). Mercure is especially useful to publish updates of resources served through web APIs in real time. It is natively supported by modern browsers (no required library nor SDK) and is very useful to update reactive web and mobile apps.
In version 2.4, I added Mercure support to the server component of API Platform and to the React and React Native app generators. The Docker Compose setup provided with API Platform has also been updated to provide a Mercure hub.
Configuring the framework to automatically dispatch updates to the currently connected clients is straightforward:
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiResource;
/**
* @ApiResource(mercure=true)
*/
class Book
{
// ...
}
Thanks to the auto-discoverability capabilities of Mercure, the generated clients will automatically subscribe to updates, and render them when received:
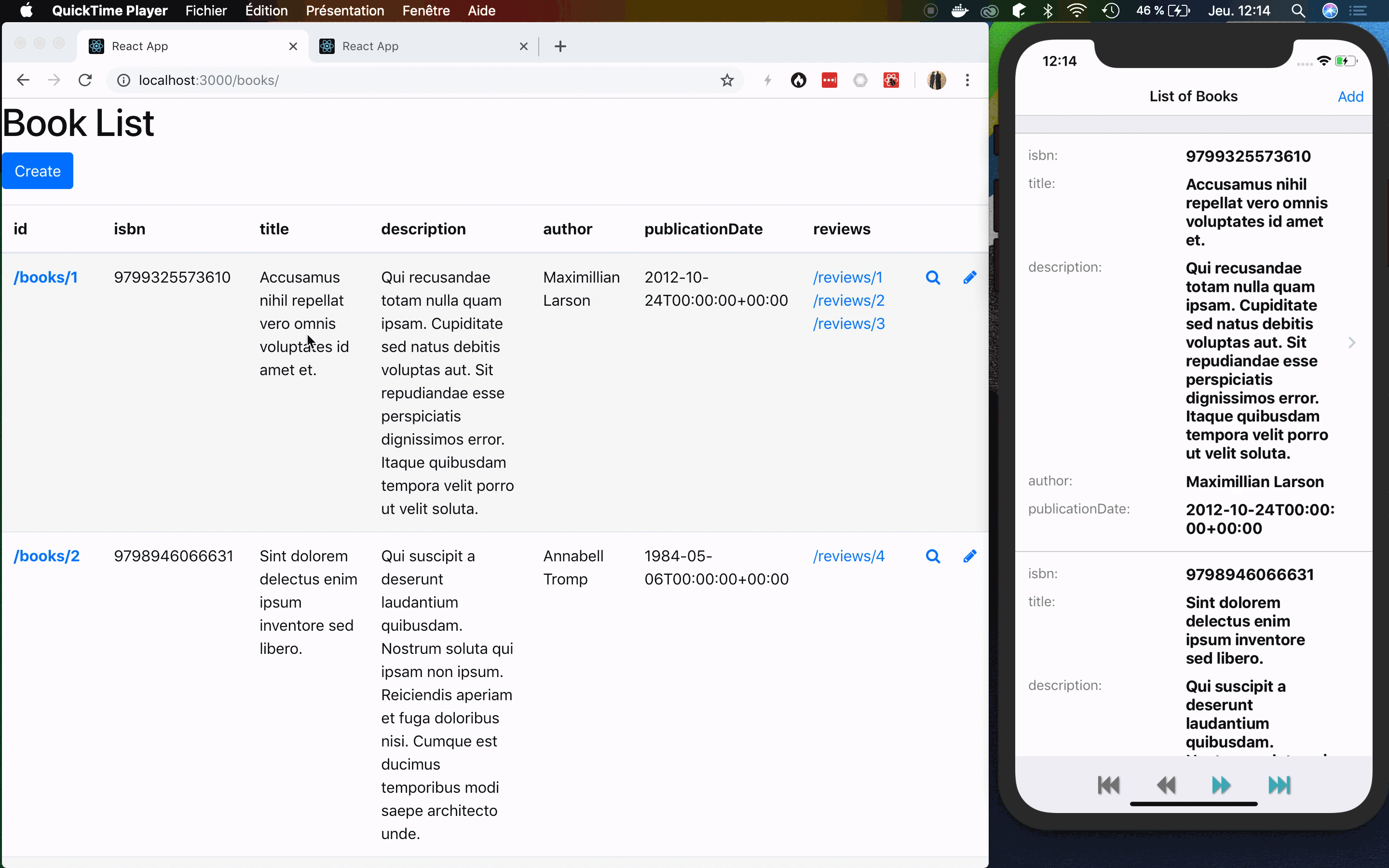
Read and improve the Mercure subsystem documentation.
CQRS and async message handling with Symfony Messenger
Messenger is a new Symfony component created by Samuel Roze (Symfony and API Platform Core Team). It allows to dispatch messages using message queues (RabbitMQ, Kafka, Amazon SQS, Google PubSub…) and to handle them asynchronously. It provides a message bus that is very useful to implement the CQRS design pattern.
In API Platform 2.4, I added a convenient way to leverage the capabilities of Messenger. This new feature is particularly useful to create service-oriented (RPC-like) endpoints:
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiResource;
use Symfony\Component\Validator\Constraints as Assert;
/**
* @ApiResource(
* messenger=true,
* collectionOperations={
* "post"={"status"=202}
* },
* itemOperations={},
* output=false
* )
*/
class ResetPasswordRequest
{
/**
* @var string
*
* @Assert\NotBlank
*/
public $username;
}
Thanks to the new messenger
attribute, this object will automatically be dispatched to the bus. It can then be handled (synchronously or asynchronously) by a message handler:
namespace App\Handler;
use App\Entity\ResetPasswordRequest;
use Symfony\Component\Messenger\Handler\MessageHandlerInterface;
class ResetPasswordRequestHandler implements MessageHandlerInterface
{
public function __invoke(ResetPasswordRequest $forgotPassword)
{
// do something with the resource
}
}
That’s all you need to use Messenger!
Read and improve the Messenger subsystem documentation.
Server Push
HTTP/2 allows a server to pre-emptively send (or “push”) responses (along with corresponding “promised” requests) to a client in association with a previous client-initiated request. This can be useful when the server knows the client will need to have those responses available in order to fully process the response to the original request.
– RFC 7540
This is capability is especially useful for REST APIs performance: it allows the server to instantly push the relations of the current resource that will be needed by the client, even before the client knows that it will have to issue an extra HTTP request.
API Platform 2.4 makes it very easy to push relations using HTTP/2:
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiProperty;
use ApiPlatform\Core\Annotation\ApiResource;
/**
* @ApiResource
*/
class Book
{
/**
* @ApiProperty(push=true)
*
* @var Author
*/
public $author;
}
Thanks to the push
attribute, if the client asks for /books/1
the web server will directly send both the requested book resource and the related author (e.g. /authors/12
) to the client.
For best performance, this feature should be used in conjunction with the built-in HTTP cache invalidation system (based on Varnish).
Read and improve the HTTP/2 Server Push subsystem documentation.
Filters detection in API Platform Admin
Jean-François Thuillier and Arnaud Oisel patched API Platform Admin and the underlying API Doc Parser JavaScript library to automatically detect and use filters exposed by the API spec (Hydra or OpenAPI). A video is worth thousand words:
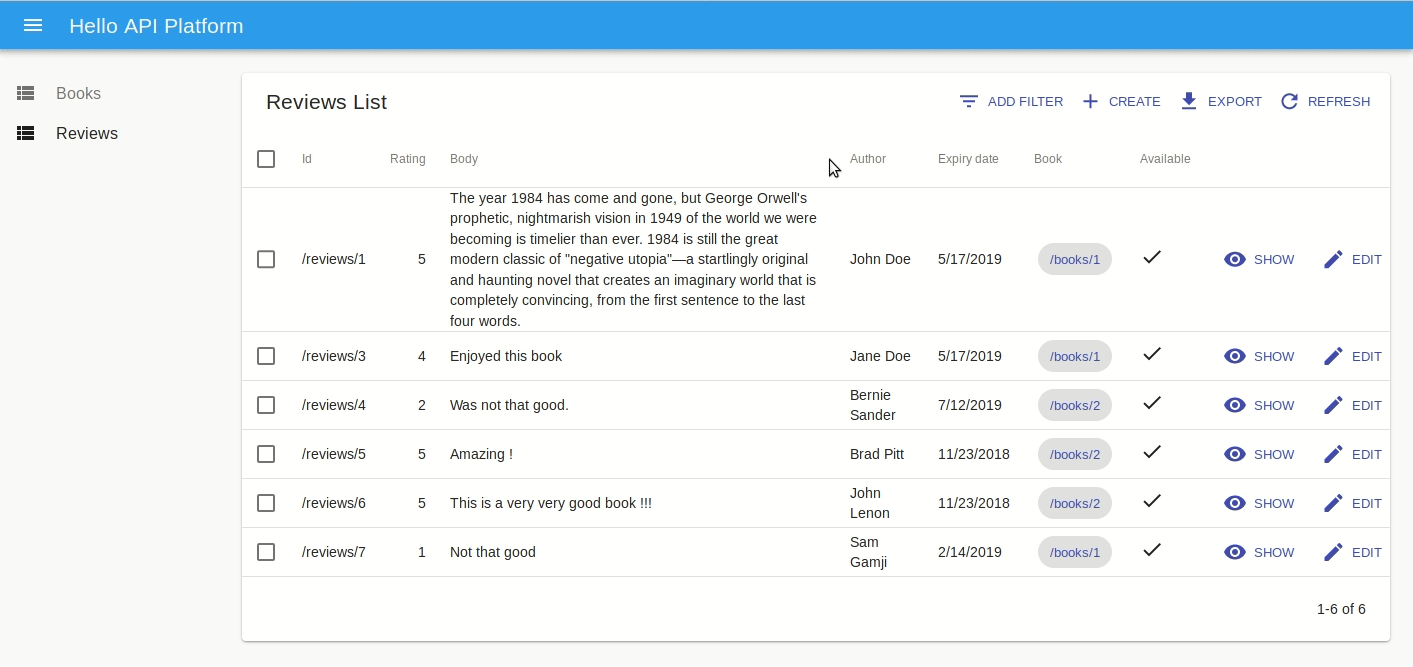
The only code that you need to get such UI is the following:
import React from 'react';
import { HydraAdmin } from '@api-platform/admin';
export default () => <HydraAdmin entrypoint="https://api.example.com"/>;
The UI is built client-side dynamically by parsing the API spec. Awesome isn’t it?
Jean-François also added some convenient helpers to help customizing the admin, and Laury Sorriaux fixed a long standing limitation: it’s now possible to use the admin even with API not served at the root of the domain (such as /api
).
Read and improve the docs of API Platform Admin.
OpenAPI v3 and ReDoc
API Platform 2.4 now fully support the new version of the OpenAPI (formerly Swagger) specification format (v3). It also leverages new features introduced by this version such as links.
To retrieve the OpenAPI specification of the API in version, use the following url: /docs.json?spec_version=3
.
You can also dump the specification in JSON or in YAML format:
bin/console api:openapi:export --spec-version=3 # JSON
bin/console api:openapi:export --spec-version=3 --yaml # YAML
Also, in addition to Swagger UI, we added support for ReDoc, a beautiful, human-readable, API docs generator written in React:
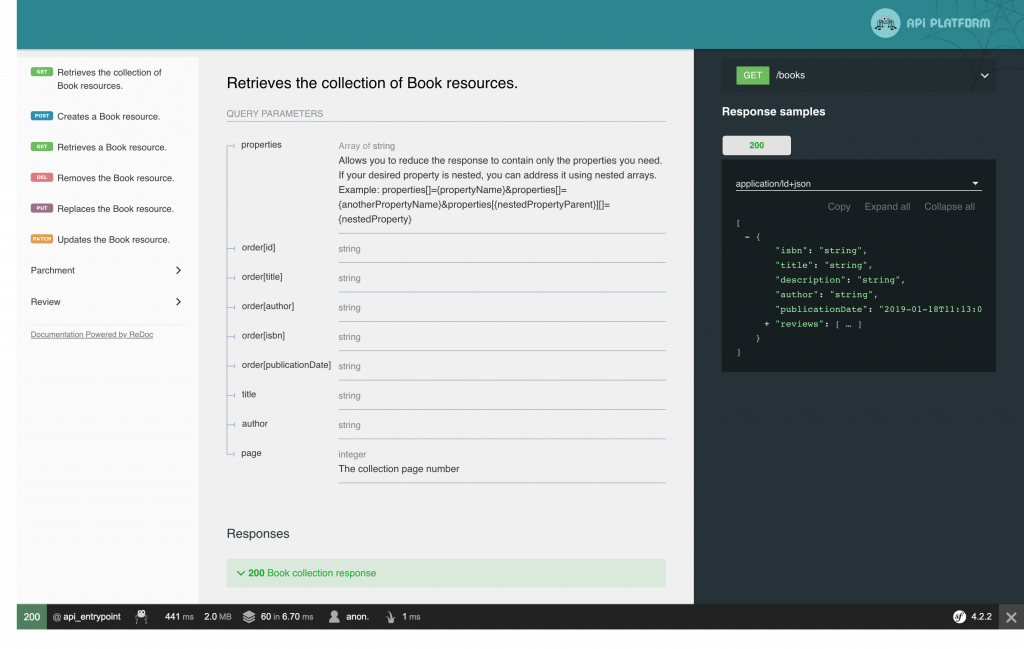
OpenAPI v3 support has been contributed by Anthony Grassiot (API Platform Core Team). ReDoc integration has been contributed by Grégoire Hébert. A big thanks to them!
Read and improve the OpenAPI related documentation.
Improved DTO support
Handling Data Transfer Objects was known to be difficult with API Platform. Back in October, the API Platform and the Sylius teams worked together to improve the API of the popular e-commerce solution… using API Platform (stay tuned!). During this workshop Antoine Bluchet and I worked on a new way to handle DTOs with API Platform:
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiResource;
use App\Dto\BookInput;
use App\Dto\BookOutput;
/**
* @ApiResource(
* input=BookInput::class,
* output=BookOutput::class
* )
*/
final class Book
{
// ...
}
The new input
and output
attributes allow to use specific classes respectively for the writeable and the readable representation of the resource.
As demonstrated in the example using Symfony Messenger, it’s also possible to set these new attributes to false
to hint that the operation will take no inputs or no outputs.
These new attributes are automatically taken into account by all API Platform subsystems, including GraphQL, Hydra and OpenAPI.
Read and improve the DTO related documentation.
Cache HTTP headers
A convenient way has been to configure the HTTP cache per resource has been added by Daniel West:
use ApiPlatform\Core\Annotation\ApiResource;
/**
* @ApiResource(cacheHeaders={"max_age"=60, "shared_max_age"=120})
*/
class Book
{
// ...
}
With this config, API Platform will automatically generate the following Cache-Control HTTP header: Cache-Control: max-age=60, public, s-maxage=120
. Thanks Daniel!
Read and improve the HTTP cache headers documentation.
Sunset HTTP header
The Sunset HTTP response header field indicates that a URI is likely to become unresponsive at a specified point in the future.
– draft-wilde-sunset-header Internet Draft
This header plays well with the deprecation mechanism available since API Platform 2.3. Thanks to a nice contribution of Thomas Blank, it’s now easy to set this header using API Platform:
namespace App\Entity;
/**
* @ApiResource(itemOperations={
* "get"={
* "deprecation_reason"="Retrieve a Book instead",
* "sunset"="01/01/2020"
* }
* })
*/
class Parchment
{
// ...
}
Read and improve the documentation related to API evolution.
Improved client generator
API Platform 2.4 is shipped with an improved version of the React and Vue.js client generators:
- Relations are now always handled properly
- HTML number fields as well as lists (arrays) are converted to the appropriate JSON type when sent to the API
- API not server at the root of the domain (such
/api
) are now supported (thanks to Fabien Kovacic)
Download and promote API Platform
API Platform 2.4 is available for download on GitHub. While you are here, if you like the project, help us making it popular by starring it on GitHub!
When will 2.4 be available through api-pack?
Hi Kevin, great work and I hope you keep things going 🙂
I’m also looking forward for the next stable release.
Any ideas as to how long we will have to wait?
How update from api-platform 2.3.6?
Pour moi et plusieurs personnes qui dépendent des APIs, Api-Platform est magistral. Ça mérite un prix… Le Turing? Bon c’est un peux gros, mais l’impact est quand même important. Bravo!
Hello All ,
By any chance will anyone have a complete api-patform docker config file with mysql instead of postgres.
To be honest, I’ve done my best to re-configure it with the version 2.4.2 it’s a nightmare !!
bunch of error cropping up and just few containers not running.
Any hint/suggestions/ideas would be much appreciated.
Thanks in advance.
Abdou